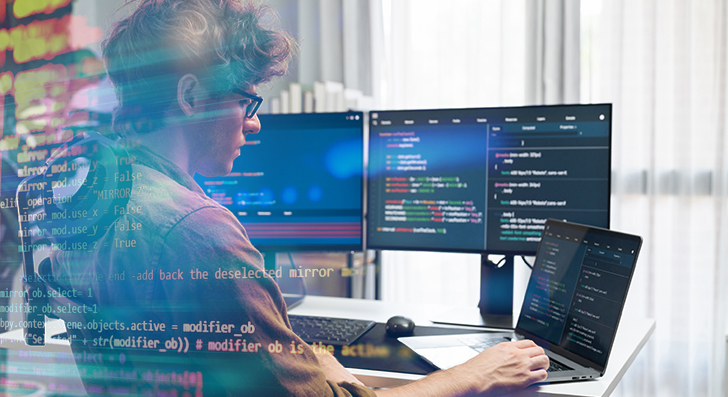
Scalability suggests your application can handle advancement—a lot more customers, more facts, plus much more targeted traffic—devoid of breaking. Like a developer, developing with scalability in your mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be part of your respective strategy from the start. Numerous applications fall short when they increase fast due to the fact the original layout can’t handle the extra load. To be a developer, you might want to Believe early regarding how your system will behave under pressure.
Start out by developing your architecture to get adaptable. Stay away from monolithic codebases exactly where anything is tightly connected. As an alternative, use modular style and design or microservices. These patterns break your app into lesser, independent elements. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it need to handle 1,000,000 end users or merely 100? Choose the correct sort—relational or NoSQL—determined by how your details will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another important stage is to prevent hardcoding assumptions. Don’t compose code that only performs under current circumstances. Take into consideration what would take place When your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design and style designs that help scaling, like message queues or occasion-driven systems. These help your application tackle extra requests with out obtaining overloaded.
Whenever you Develop with scalability in your mind, you are not just planning for achievement—you are reducing future head aches. A well-prepared process is simpler to maintain, adapt, and grow. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Picking out the proper database is usually a critical Section of developing scalable applications. Not all databases are designed precisely the same, and using the Incorrect you can sluggish you down or even induce failures as your app grows.
Start by being familiar with your knowledge. Is it remarkably structured, like rows within a table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally strong with associations, transactions, and consistency. Additionally they assistance scaling approaches like examine replicas, indexing, and partitioning to deal with extra site visitors and details.
When your facts is more versatile—like person action logs, product or service catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, consider your read through and create designs. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be managing a hefty publish load? Take a look at databases that may tackle higher publish throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for non permanent information streams).
It’s also wise to Consider ahead. You may not require Innovative scaling capabilities now, but deciding on a database that supports them signifies you received’t have to have to modify later.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor databases performance as you grow.
In short, the correct database is determined by your app’s structure, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly penned code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s essential to Create effective logic from the beginning.
Commence by creating clean up, very simple code. Keep away from repeating logic and remove everything needless. Don’t pick the most advanced Remedy if a simple just one performs. Keep your capabilities quick, focused, and simple to test. Use profiling tools to uncover bottlenecks—spots exactly where your code usually takes way too long to operate or utilizes too much memory.
Following, take a look at your databases queries. These frequently slow factors down greater than the code by itself. Make certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches every thing, and as a substitute decide on unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, In particular throughout huge tables.
For those who discover precisely the same data getting asked for over and over, use caching. Retail outlet the results briefly working with applications like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your database operations any time you can. Instead of updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could possibly crash when they have to handle one million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more people plus much more website traffic. If all the things goes as a result of one server, it will rapidly become a bottleneck. That’s where load balancing and caching are available. These two tools help keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across multiple servers. Instead of one server accomplishing many of the get the job done, the load balancer routes end users to distinctive servers based upon availability. What this means is no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused immediately. When people request the same facts once again—like an item website page or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching cuts down database load, increases pace, and will make your app additional effective.
Use caching for things which don’t change typically. And always be sure your cache is current when info does improve.
In brief, load balancing and caching are uncomplicated but potent equipment. Alongside one another, they help your application handle a lot more buyers, stay quickly, and Get well from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need tools that let your app increase quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t must get components or guess long run ability. When targeted visitors improves, you can add more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide companies like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This can make it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application works by using a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect within your app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, utilizing cloud and container applications implies you could scale rapidly, deploy easily, and Get better swiftly when complications take place. If you prefer your app to improve with out boundaries, start employing these applications early. They conserve time, lower risk, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, spot problems early, and make greater conclusions as your application grows. It’s a key Portion of making scalable check here units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this data.
Don’t just keep track of your servers—check your app also. Control just how long it will require for people to load web pages, how frequently glitches transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you resolve problems fast, normally right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh attribute and see a spike in faults or slowdowns, you may roll it back again before it results in authentic injury.
As your application grows, website traffic and info increase. Without the need of monitoring, you’ll miss indications of difficulty until it’s far too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant organizations. Even small apps have to have a powerful Basis. By designing meticulously, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking under pressure. Start out small, Consider significant, and Develop sensible.